Okay, so I found myself needing to make my Arduino control something a bit hefty the other day. We’re talking about stuff that needs more juice than those little Arduino pins can safely push out, like a decent-sized DC motor or maybe a long strip of LEDs.
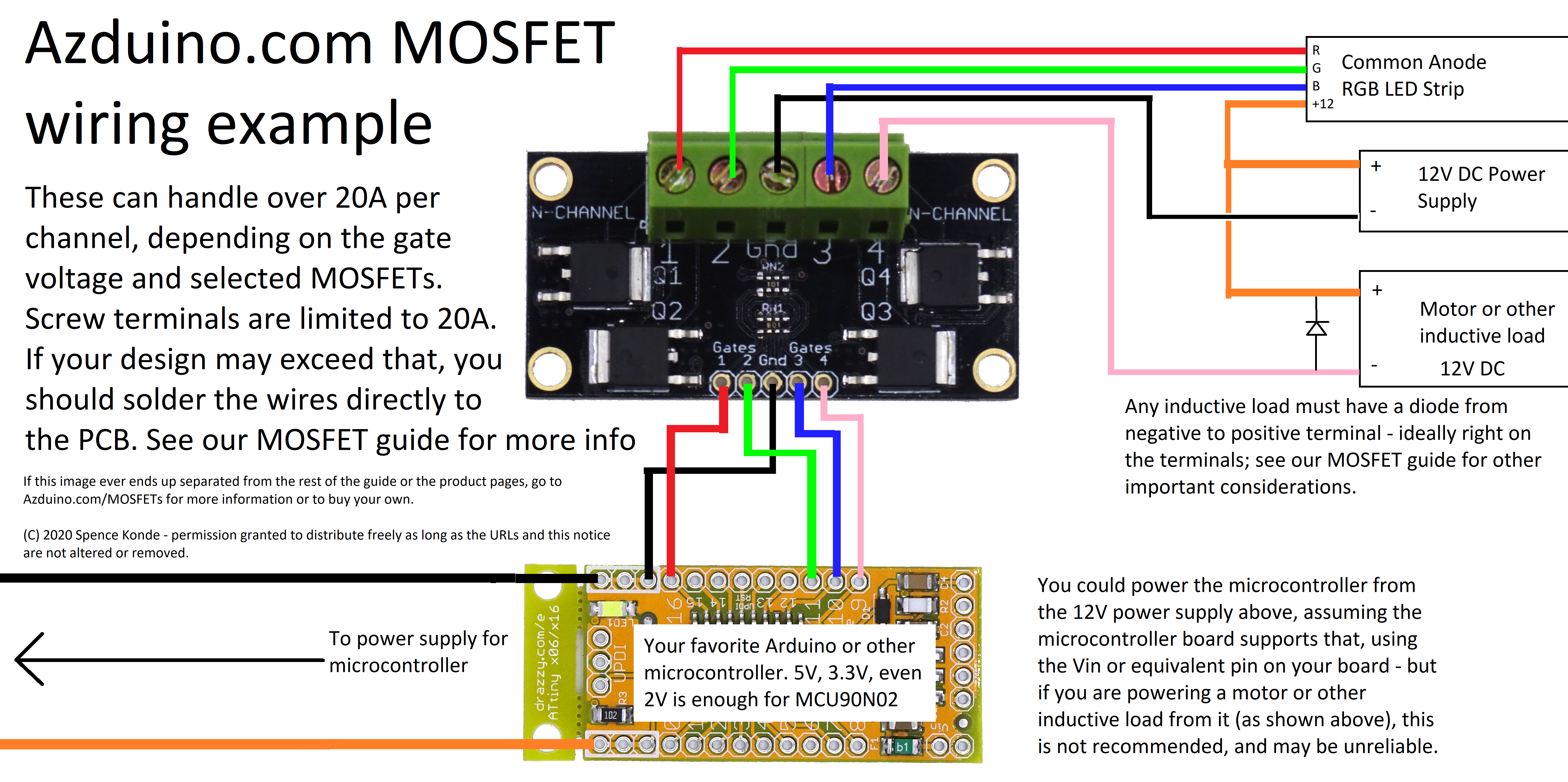
First thing I realized, pretty quickly, was that you just can’t hook up that kind of load directly to an Arduino output pin. Those pins are rated for maybe 40 milliamps max, and at 5 volts. Trying to run a 12V motor or a bunch of LEDs that pull amps? Yeah, that’s a recipe for a fried Arduino chip. Learned that the hard way a long time ago.
So, I needed a way to switch the bigger power using the small signal from the Arduino. My mind immediately went to MOSFETs. I’ve used relays before, but MOSFETs are solid-state, faster, and generally quieter. They act like an electronic gatekeeper – the Arduino sends a tiny signal to the ‘gate’ pin, which then opens or closes the main path for the big current to flow between the ‘source’ and ‘drain’ pins.
Getting Started with the Hookup
I rummaged through my component drawers and found some N-Channel MOSFETs. It’s important to grab ones that are “logic level” if you’re driving them straight from an Arduino. This just means the MOSFET will turn fully on with the 5 volts the Arduino provides at its gate pin. If you use a non-logic-level one, it might not turn on completely, or at all, and it might get really hot.
Wiring it up was the next step. Here’s how I typically do it for controlling a load like a motor with a separate power supply (say, 12V):
- The MOSFET’s Gate pin goes to a digital output pin on the Arduino.
- The MOSFET’s Source pin goes to Ground (GND). Very important: This ground needs to be connected to both the Arduino’s GND and the negative terminal of your separate 12V power supply. Common ground is key!
- The MOSFET’s Drain pin connects to the negative lead of the motor (or LED strip, etc.).
- The positive lead of the motor connects to the positive terminal of the 12V power supply.
I also remembered from past fiddling that it’s a good idea to put a pull-down resistor (something like 10K ohms) between the MOSFET’s Gate pin and Ground. This makes sure the MOSFET stays firmly off when the Arduino pin isn’t actively pulling it high, or maybe during startup when the pin state is undefined. Without it, the gate can sometimes ‘float’ and the MOSFET might turn on or off unexpectedly.
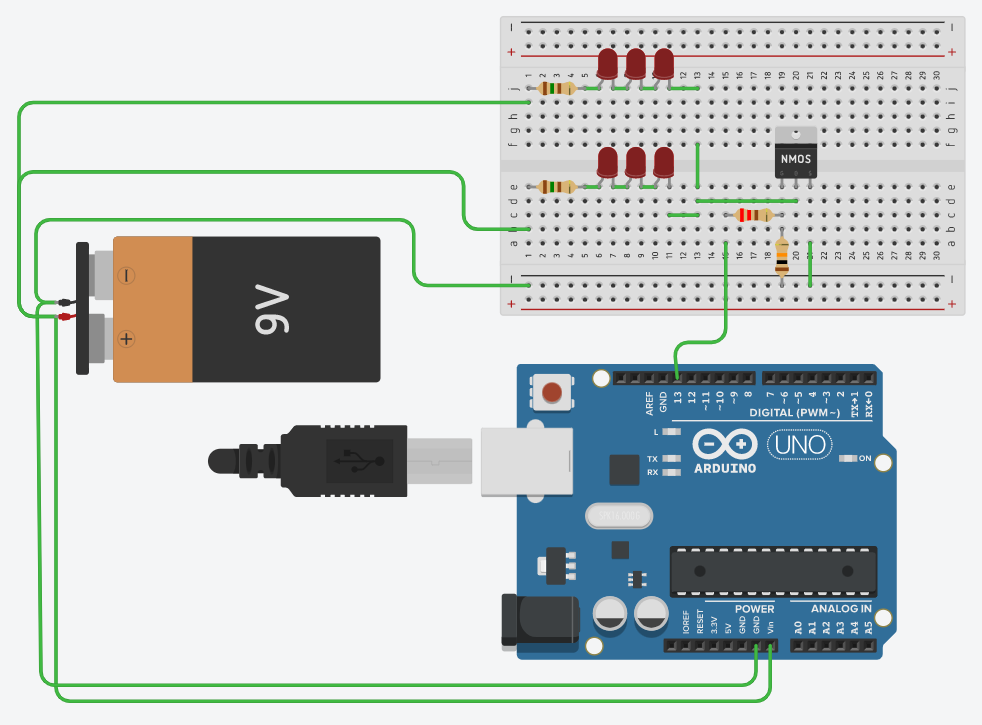
Coding and Testing Time
With the wiring sorted, I wrote a super simple sketch on the Arduino. Basically just using `pinMode()` to set the connected pin as an OUTPUT, and then `digitalWrite()` to turn it HIGH or LOW.
// Super simple example – not real code, just the idea
// int mosfetPin = 9; // Arduino pin connected to MOSFET Gate
// void setup() {
// pinMode(mosfetPin, OUTPUT);
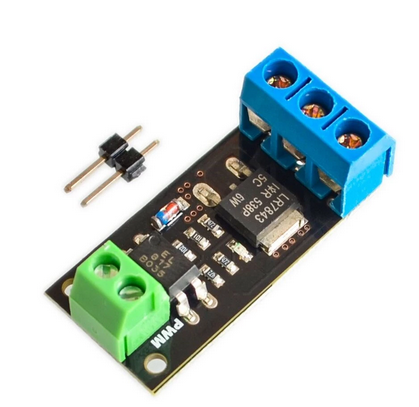
// }
// void loop() {
// digitalWrite(mosfetPin, HIGH); // Turn MOSFET ON
// delay(1000);
// digitalWrite(mosfetPin, LOW); // Turn MOSFET OFF
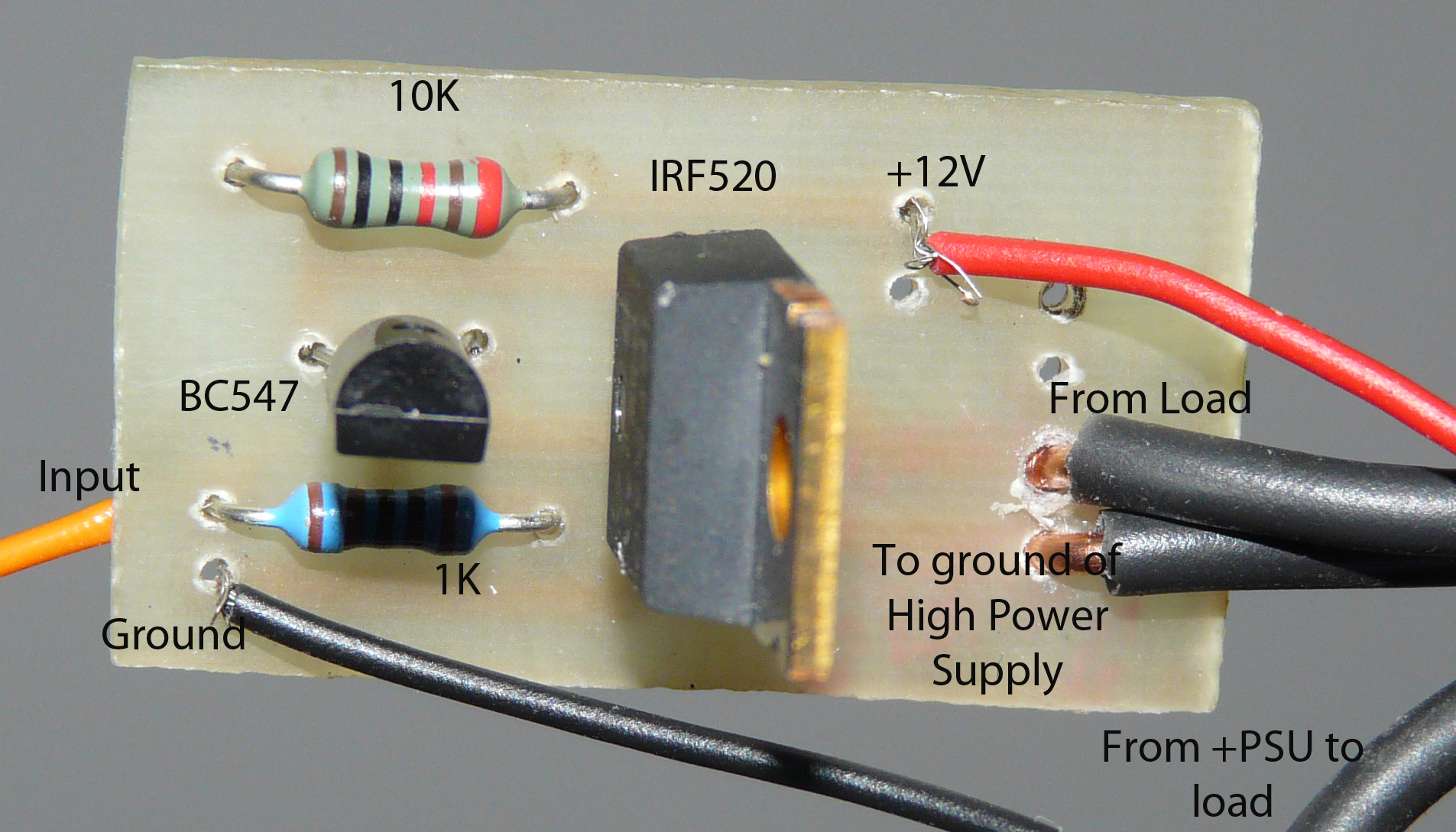
// delay(1000);
// }
Uploaded the code, held my breath for a second (you always do, right?), and powered everything up. Success! The motor turned on when `digitalWrite` was HIGH and turned off when it was LOW. The MOSFET handled the 12V motor current without bothering the Arduino.
Taking it Further with PWM
Just on/off is fine, but often you want variable control, like changing motor speed or LED brightness. That’s easy too. I just made sure the Arduino pin connected to the Gate was one of the PWM-capable pins (usually marked with a ‘~’ symbol). Then, instead of `digitalWrite()`, I used `analogWrite(pin, value)`, where `value` can be anything from 0 (fully off) to 255 (fully on). Using values in between gives you proportional control. Worked like a charm for dimming the LEDs I tested next.
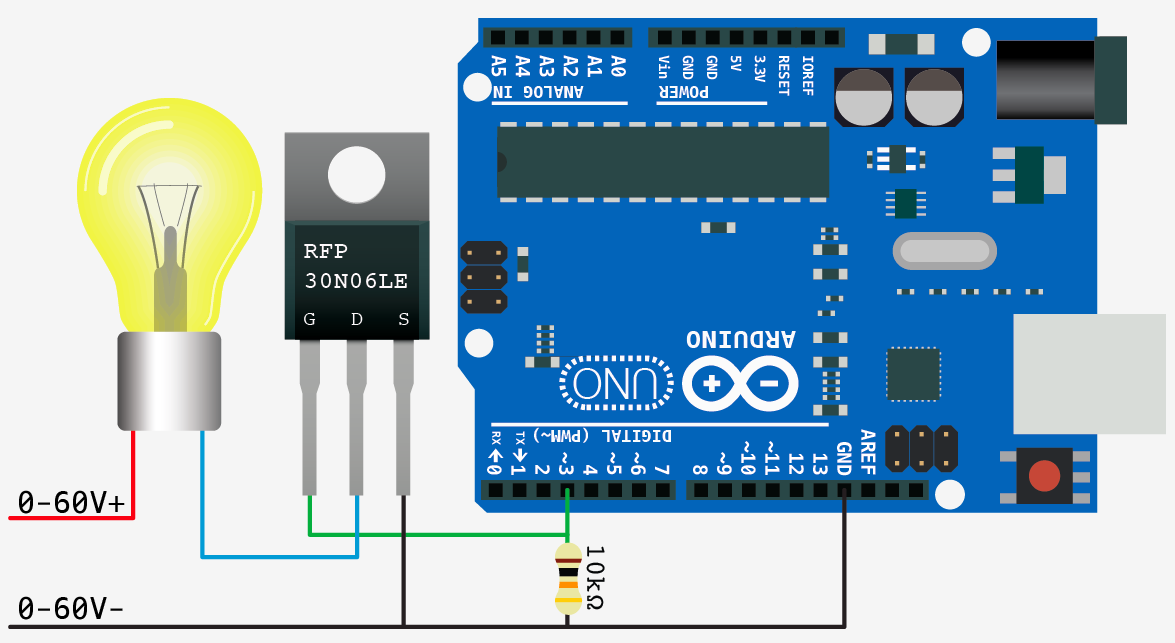
One thing I always keep an eye on is heat. If you’re switching a really heavy load, or switching very fast with PWM, the MOSFET can get hot. If it gets too hot to touch comfortably, it’s definitely time to screw on a heatsink to help it dissipate that heat.
So, that’s my basic process for using MOSFETs with Arduino. It’s a really fundamental technique for bridging the gap between the low-power microcontroller world and higher-power devices. Once you get the hang of it, it opens up a ton of possibilities for projects.