Okay, so yesterday I was messing around with MOSFETs, trying to drive a small motor. Figured I’d document the whole thing ’cause, why not? Maybe someone else will find it useful when they’re scratching their heads.
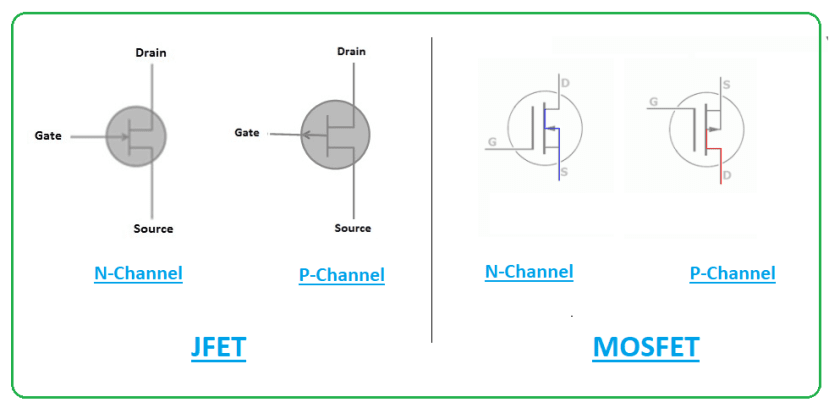
First off, I grabbed a handful of MOSFETs I had lying around. Ended up using an IRLB8721. Seemed beefy enough for what I was doing. I always check the datasheet first, just to make sure I’m not gonna blow anything up. Found it online, you should always do the same.
The Circuit
- Power supply (12V in this case)
- The IRLB8721 MOSFET
- A small DC motor
- A resistor (1k ohm)
- Some jumper wires, breadboard – you know, the usual stuff.
I started by hooking up the motor directly to the power supply. Just to see it spin and make sure it actually worked. It did, so that was a good start.
Then, I built the circuit on the breadboard. Here’s roughly how it went:
- Connected the drain of the MOSFET to one terminal of the motor.
- Connected the other terminal of the motor to the 12V power supply.
- Connected the source of the MOSFET to ground.
- Connected the 1k resistor between the gate of the MOSFET and ground (to make sure the gate isn’t floating).
- Then, here’s where the magic happens – I used a jumper wire from my microcontroller (an Arduino, but anything that can output 5V should work) to the gate of the MOSFET. This is what turns the MOSFET on and off.
At first, nothing happened. I was like, “Uh oh.” Checked all my connections, made sure everything was plugged in correctly. Still nothing.
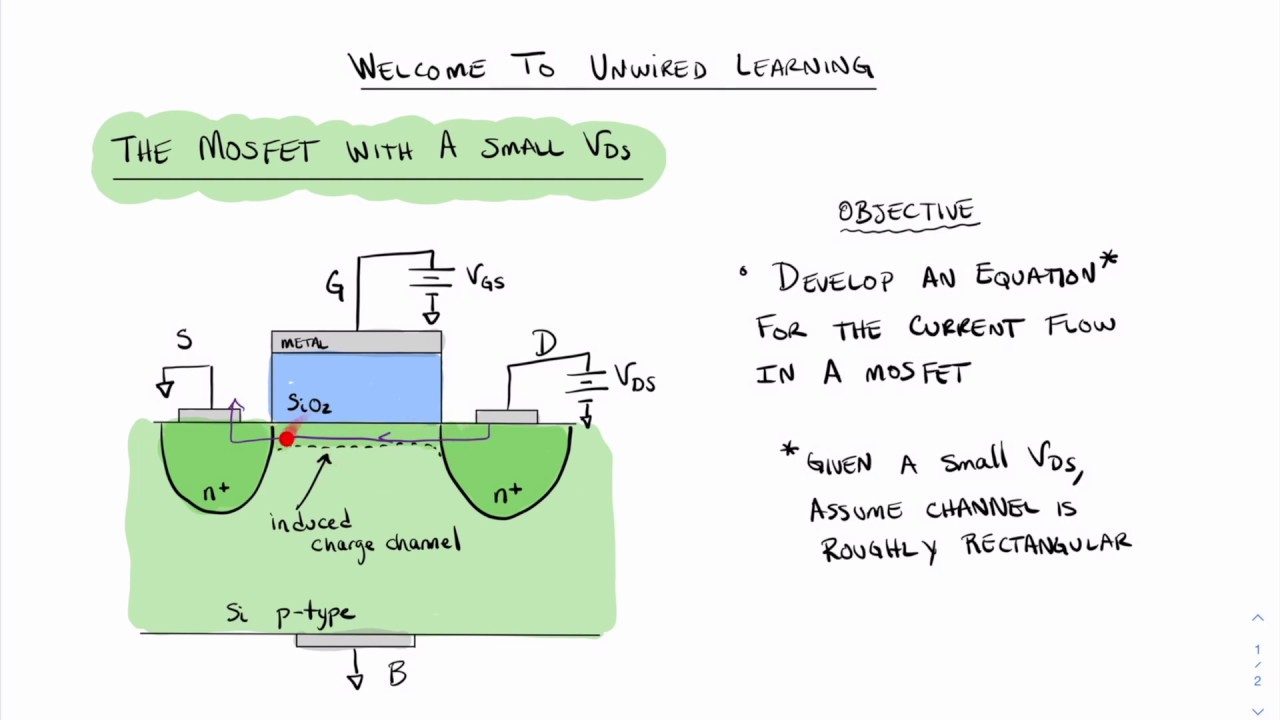
Then I remembered: the gate needs enough voltage to actually turn the MOSFET ON! The IRLB8721 is a logic-level MOSFET, which means it should turn on fully with 5V. But maybe the voltage from my Arduino wasn’t quite high enough, or maybe the MOSFET needed a little more of a kick.
So, I tried connecting the gate directly to the 5V pin on the Arduino (bypassing any code or anything). Still nothing! I was getting kinda frustrated at this point.
I then remembered I had a multimeter. First I checked the voltage to make sure my power supply was outputting the correct voltage, and that my arduino was outputting 5V. All checked out ok.
The key thing I was missing was to check the voltage between the gate and the source (Vgs). So I measured the voltage between the gate and the source while the “on” signal was applied from the Arduino. It was only 4.5V. That’s why it wouldn’t turn on all the way. I tried a different Arduino board that was giving a slightly higher voltage and BAM! Success.
Once I got that sorted, I wrote a quick Arduino sketch to turn the motor on and off every second. Just to see it working. It was pretty simple stuff:
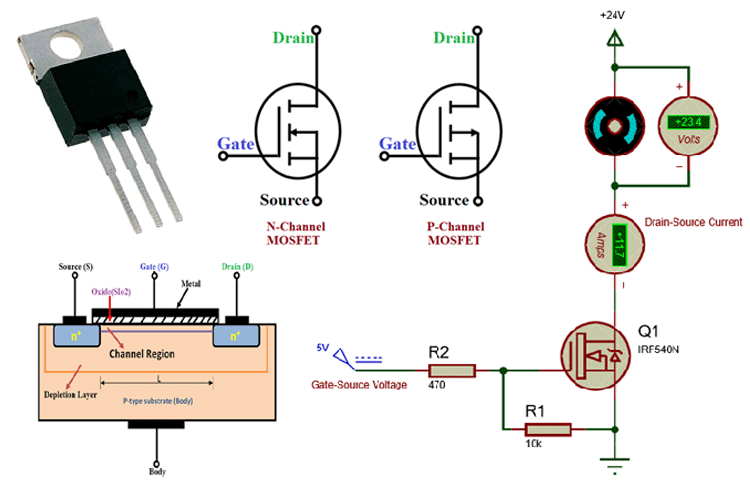
c++
int motorPin = 8; // Connect the gate of the MOSFET to digital pin 8
void setup() {
pinMode(motorPin, OUTPUT);
void loop() {
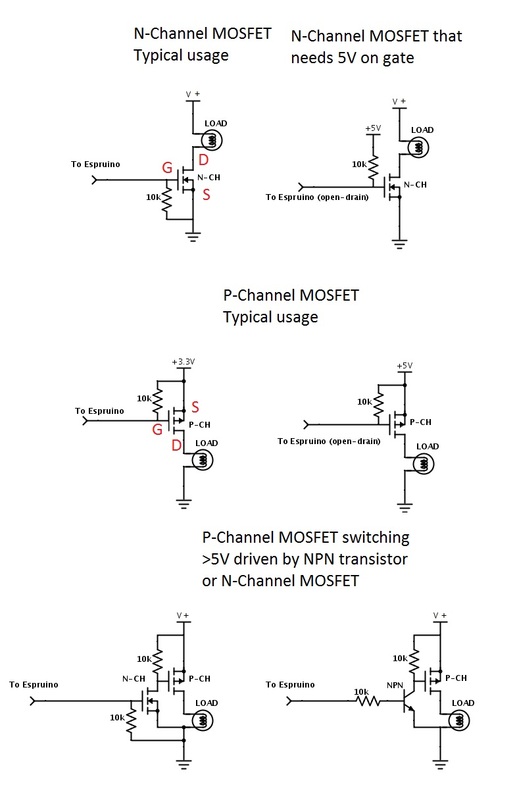
digitalWrite(motorPin, HIGH); // Turn the motor on
delay(1000);
digitalWrite(motorPin, LOW); // Turn the motor off
delay(1000);
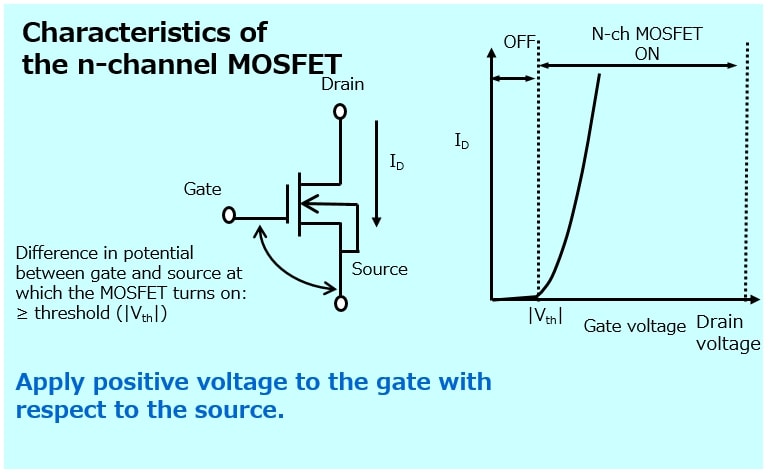
Uploaded the code to the Arduino, and the motor started spinning on and off like clockwork. Pretty cool!
Learnings
- Always double-check your connections. Seriously, 90% of the time, it’s just a loose wire or something stupid like that.
- Logic-level MOSFETs are great, but still double-check the datasheet to make sure the voltage from your microcontroller is enough to fully turn them on.
- Multimeters are your friend. Use them!
It was a fun little project. Next time, I’m thinking of trying to control the speed of the motor using PWM (Pulse Width Modulation). That should be interesting. I’ll keep you posted!